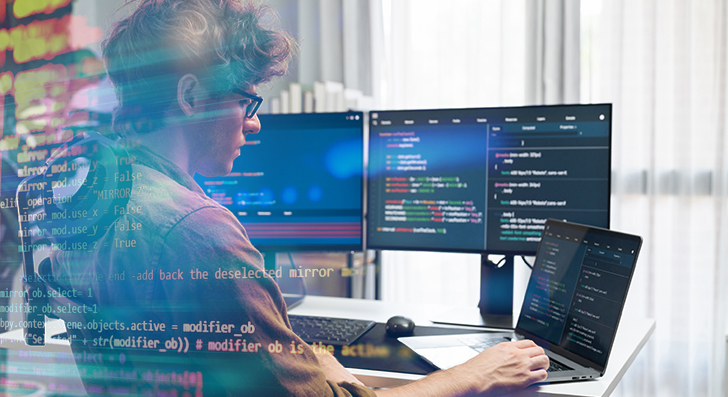
Scalability implies your software can take care of progress—much more users, additional knowledge, and a lot more visitors—without breaking. To be a developer, constructing with scalability in mind will save time and anxiety afterwards. Listed here’s a clear and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be section of the plan from the beginning. Lots of apps are unsuccessful whenever they grow rapidly due to the fact the original layout can’t handle the extra load. To be a developer, you should Imagine early about how your process will behave under pressure.
Get started by developing your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. Rather, use modular layout or microservices. These styles split your application into smaller, independent areas. Each module or services can scale By itself devoid of affecting The full procedure.
Also, consider your database from working day just one. Will it need to deal with 1,000,000 end users or simply just a hundred? Select the suitable style—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t have to have them however.
A different vital point is to avoid hardcoding assumptions. Don’t create code that only operates beneath recent ailments. Contemplate what would materialize Should your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use structure styles that guidance scaling, like information queues or celebration-driven units. These assistance your application cope with additional requests devoid of receiving overloaded.
If you Establish with scalability in mind, you are not just planning for achievement—you are decreasing long term headaches. A well-prepared process is simpler to maintain, adapt, and grow. It’s better to arrange early than to rebuild later.
Use the Right Databases
Picking out the proper database is usually a critical Section of constructing scalable applications. Not all databases are created precisely the same, and using the wrong you can sluggish you down or even bring about failures as your app grows.
Start by being familiar with your knowledge. Could it be extremely structured, like rows inside of a table? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are generally powerful with interactions, transactions, and consistency. They also guidance scaling strategies like browse replicas, indexing, and partitioning to deal with extra targeted traffic and information.
If the information is much more adaptable—like person activity logs, product or service catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured details and can scale horizontally far more very easily.
Also, take into consideration your go through and produce styles. Have you been accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you handling a weighty create load? Investigate databases which can deal with large produce throughput, or even occasion-based mostly facts storage units like Apache Kafka (for temporary facts streams).
It’s also smart to Believe ahead. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t want to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your data based on your accessibility patterns. And usually check database efficiency when you mature.
To put it briefly, the ideal databases relies on your application’s framework, pace demands, And the way you count on it to expand. Acquire time to choose correctly—it’ll preserve plenty of difficulty later.
Improve Code and Queries
Speedy code is vital to scalability. As your app grows, each little delay adds up. Improperly published code or unoptimized queries can slow down overall performance and overload your method. That’s why it’s important to Establish successful logic from the start.
Start by crafting cleanse, very simple code. Prevent repeating logic and remove something avoidable. Don’t select the most sophisticated solution if a straightforward a single works. Keep the features short, focused, and simple to test. Use profiling applications to seek out bottlenecks—locations where by your code normally takes too very long to run or takes advantage of excessive memory.
Following, take a look at your databases queries. These frequently sluggish issues down in excess of the code itself. Ensure that Each and every question only asks for the data you really need. Keep away from Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And stay away from executing too many joins, Specially throughout big tables.
In case you notice the exact same information currently being asked for again and again, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra effective.
Remember to examination with substantial datasets. Code and queries that do the job good with 100 data could possibly crash when they have to deal with 1 million.
In brief, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software continue to be easy and responsive, whilst the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with much more consumers and even more targeted traffic. If almost everything goes by way of just one server, it can promptly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to a single server carrying out every one of the operate, the load balancer routes consumers to various servers according to availability. This suggests no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to setup.
Caching is about storing info temporarily so it might be reused swiftly. When customers ask for precisely the same info all over again—like a product page or simply a profile—you don’t ought to fetch it in the databases each and every time. You'll be able to provide it through the cache.
There are two prevalent varieties of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for fast entry.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the user.
Caching lessens database load, enhances velocity, and can make your application a lot more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does adjust.
In a nutshell, load balancing and caching are very simple but potent instruments. Together, they help your application tackle a lot more people, stay quickly, and Get well from problems. If you plan to increase, you need each.
Use Cloud and Container Tools
To construct scalable apps, you would like tools that let your app increase conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to rent servers and solutions as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors increases, you are able to include much more sources with only a few clicks or instantly making use of automobile-scaling. When website traffic drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection instruments. You may center on constructing your app as opposed to handling infrastructure.
Containers are An additional key Software. A container offers your app and every little thing it must run—code, libraries, settings—into a person device. This causes it to be simple to maneuver your application among environments, out of your notebook to your cloud, with no surprises. Docker is the most popular tool for this.
Once your app uses various containers, instruments like Kubernetes enable you to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it instantly.
Containers also enable it to be simple to separate portions of your app into products and services. It is possible to update or scale parts independently, and that is great for general performance and dependability.
In short, employing cloud and container tools means you may scale quick, deploy quickly, and recover promptly when issues transpire. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease chance, and help you remain centered on creating, not correcting.
Monitor Almost everything
For those who don’t keep track of your application, you received’t know when matters go Improper. Checking can help the thing is how your app is executing, place challenges early, and make much better choices as your application grows. It’s a critical part of developing scalable programs.
Begin by tracking standard metrics like CPU utilization, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just keep track of your servers—keep track of your app also. Keep watch over just how long it requires for end users to load web pages, how frequently glitches transpire, and where they happen. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s happening inside your code.
Set up alerts for important problems. For example, if your reaction time goes higher than a Restrict or maybe a assistance goes down, you must get notified quickly. This will help you resolve problems quick, often right before buyers even detect.
Monitoring can be valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and read more knowledge improve. Without the need of checking, you’ll miss indications of problems until it’s far too late. But with the correct tools in position, you remain on top of things.
In a nutshell, monitoring will help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about knowledge your program and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even little applications need a powerful Basis. By creating thoroughly, optimizing wisely, and utilizing the ideal resources, you are able to Make applications that expand efficiently with out breaking under pressure. Get started little, Consider big, and Construct clever.